Quick Cyber Thoughts: Tuw GUI Wrapper
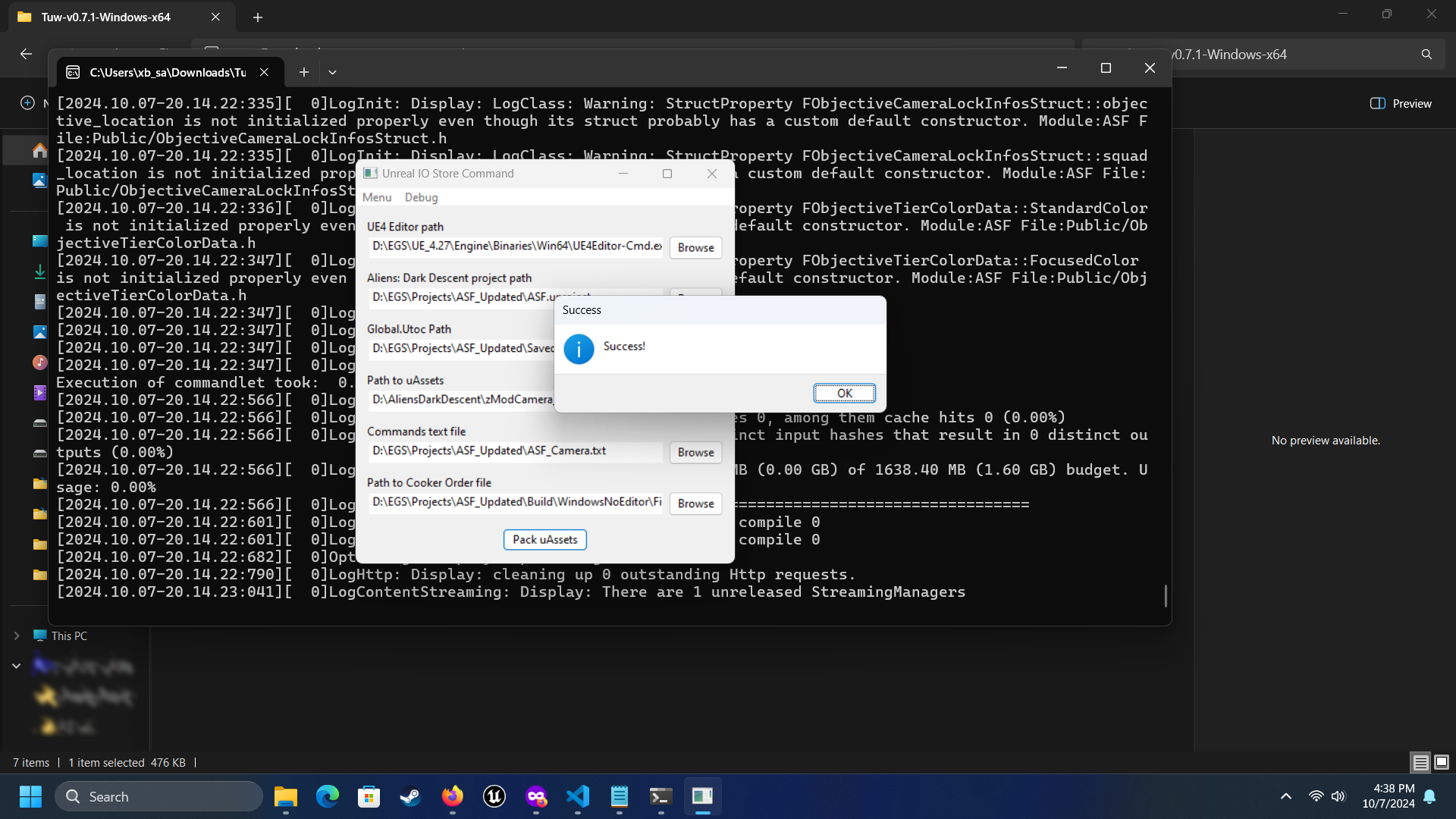
I have a love-hate relationship with command line.
On one hand, I cannot deny the speed and efficiency command line has with some tasks.
On the other, command line requires not only knowledge of the commands and variables to input, but it requires a lot of typing, copy-pasting, and/or trial and error to get working properly.
Now, AI can make the iteration process a lot easier and faster, and once you've gotten a command perfected, you can make it into a script file, but most people are familiar and more comfortable with a Graphical User Interface (GUI).
But what if you could come up with a GUI for your Command Line Interface (CLI) commands?
Tuw - The Open Source Solution
I discovered Tuw thanks to someone on the Unreal Engine Modding Discord. (I would give credit, but it's very annoying to search Discord for things like this.)
A large number of tools for modding Unreal Engine games are CLI tools, which often involve multiple file paths and variables, and generally don't have a GUI created by the actual tool developer. Also, the documentation on the Github pages tend not to be the best. So they tend to be incredibly unfriendly to new users, who tend to search around for knowledge on how to mod a specific game that happens to use Unreal Engine.
How Tuw works is pretty simple. There is an executable file (.EXE for Windows, and other formats for Mac and Linux) that checks a JSON file for the following things:
- The actual command.
- A button to execute the command.
- Input fields.
- Labels for the fields and the button.
- Identifiers for variables
- Rules for input validation and error handling.
- A wide variety of other settings.
Keep It Stupid Simple
There is an old axiom that you may have heard of: KISS.
You may be familiar with what it usually spells out: Keep It Simple, Stupid. It's a warning to keep people from creating overcomplicated solutions, because people like to associate complexity with sophistication. But there's a flip side of the coin that people tend to ignore.
Keep It Stupid Simple is an informal design goal. The polite summation is that the solution being designed for the task should be as simple and easy as possible, so that even an uneducated person can do the job. Some would say that Apple is a master of this, but other relevant (but non-tech related) examples would be the AK and Glock. If the thing you design can be easily and successfully used by anyone within a half hour or less, it's stupid simple.
Tuw very much fits this mold. Let's look at the JSON code I created to handle the task:
{
"gui": [
{
"label": "Minimal Sample",
"window_name": "Unreal IO Store Command",
"command": "%UE4editor% %uProject% -run=IoStore -CreateGlobalContainer=%GlobalContainer% -CookedDirectory=%CookedDir% -Commands=%Commands% -CookerOrder=%CookerOrder% -TargetPlatform=WindowsNoEditor -stdout -UTF8Output",
"button": "Pack uAssets",
"components": [
{
"type": "file",
"label": "UE4 Editor path",
"extension": "EXE files (*.exe)|*.exe",
"placeholder": "D:\\EGS\\UE_4.27\\Engine\\Binaries\\Win64\\UE4Editor-Cmd.exe",
"id": "UE4editor",
"validator": {
"exist": true,
"not_empty": true,
"wildcard": "*.exe"
}
},
{
"type": "file",
"label": "Aliens: Dark Descent project path",
"extension": "uProject files (*.uproject)|*.uproject",
"placeholder": "D:\\EGS\\Projects\\ASF_Updated\\ASF.uproject",
"id": "uProject",
"validator": {
"exist": true,
"not_empty": true,
"wildcard": "*.uproject"
}
},
{
"type": "file",
"label": "Global.Utoc Path",
"extension": "uTOC files (*.utoc)|*.utoc",
"placeholder": "D:\\EGS\\Projects\\ASF_Updated\\Saved\\StagedBuilds\\WindowsNoEditor\\ASF\\Content\\Paks\\global.utoc",
"id": "GlobalContainer",
"validator": {
"exist": true,
"not_empty": true,
"wildcard": "*.utoc"
}
},
{
"type": "folder",
"label": "Path to uAssets",
"placeholder": "D:\\AliensDarkDescent\\zModCamera",
"add_quotes": false,
"id": "CookedDir"
},
{
"type": "file",
"label": "Commands text file",
"extension": "Text files (*.txt)|*.txt",
"placeholder": "D:\\EGS\\Projects\\ASF_Updated\\ASF_Upgrades.txt",
"add_quotes": true,
"id": "Commands",
"validator": {
"exist": true,
"not_empty": true,
"wildcard": "*.txt"
}
},
{
"type": "file",
"label": "Path to Cooker Order file",
"extension": "Log files (*.log)|*.log",
"placeholder": "D:\\EGS\\Projects\\ASF_Updated\\Build\\WindowsNoEditor\\FileOpenOrder\\CookerOpenOrder.log",
"add_quotes": false,
"id": "CookerOrder",
"validator": {
"exist": true,
"not_empty": true,
"wildcard": "*.log"
}
}
]
}
]
}
Tuw comes with extensive documentation on how to set up the JSON file. And most crucially, it comes with example code that actually shows how the code should be configured and integrated with the other code. A great deal of documentation for other items presupposes that the user has a great deal of applicable knowledge going in... Which then causes problems when the user does not have that knowledge.
And modding is perhaps the quintessential example of that type of scenario. In most circumstances, the person who wants to mod has zero experience with the specific programs they have to use. They might be familiar with editing code or configuration files, but perhaps not command line interfaces, especially if they're coming from a game where there's an official mod tool kit.
(I speak from experience.)
The Tuw GUI Crafting Experience
Making a GUI with Tuw is quite fast. Between copying and pasting code straight from the Github documentation and auto-complete, the main time sink will be the complexity of the command and the GUI. More fields naturally increases the amount of time spent making the GUI, especially if they're of multiple different types and require different types of validation.
Testing is a bit finicky, because the program expects you to use gui_definition.json
as the file name. So there's no way to switch between GUIs without coding 2+ GUIs' worth of JSON code. This is a massive inconvenience if you plan to make a Tuw program with the JSON code embedded in it for distribution.
(The issue is that because Tuw defaults to reading gui_definition.json
, which is the file that can be set to allow Tuw to generate a new version of Tuw with a GUI definition embedded in it. So have to have two separate JSONs, and the new EXE will have an annoying popup on run alerting you to the fact that it's ignoring gui_definition.json
in favor of the one you specified.)
Once you initialize Tuw, go through the steps required to fill out the various fields, then press the button to execute the command. If there's an error, it'll show up if you have validator or error handling code. In my case, I initially had regular expression and wildcard matching for the file extensions, but for some reason, every single file reported as invalid. Removing those lines from the JSON fixed the problem.
Once that was done, I went through the process of filling out the fields and got the following result:
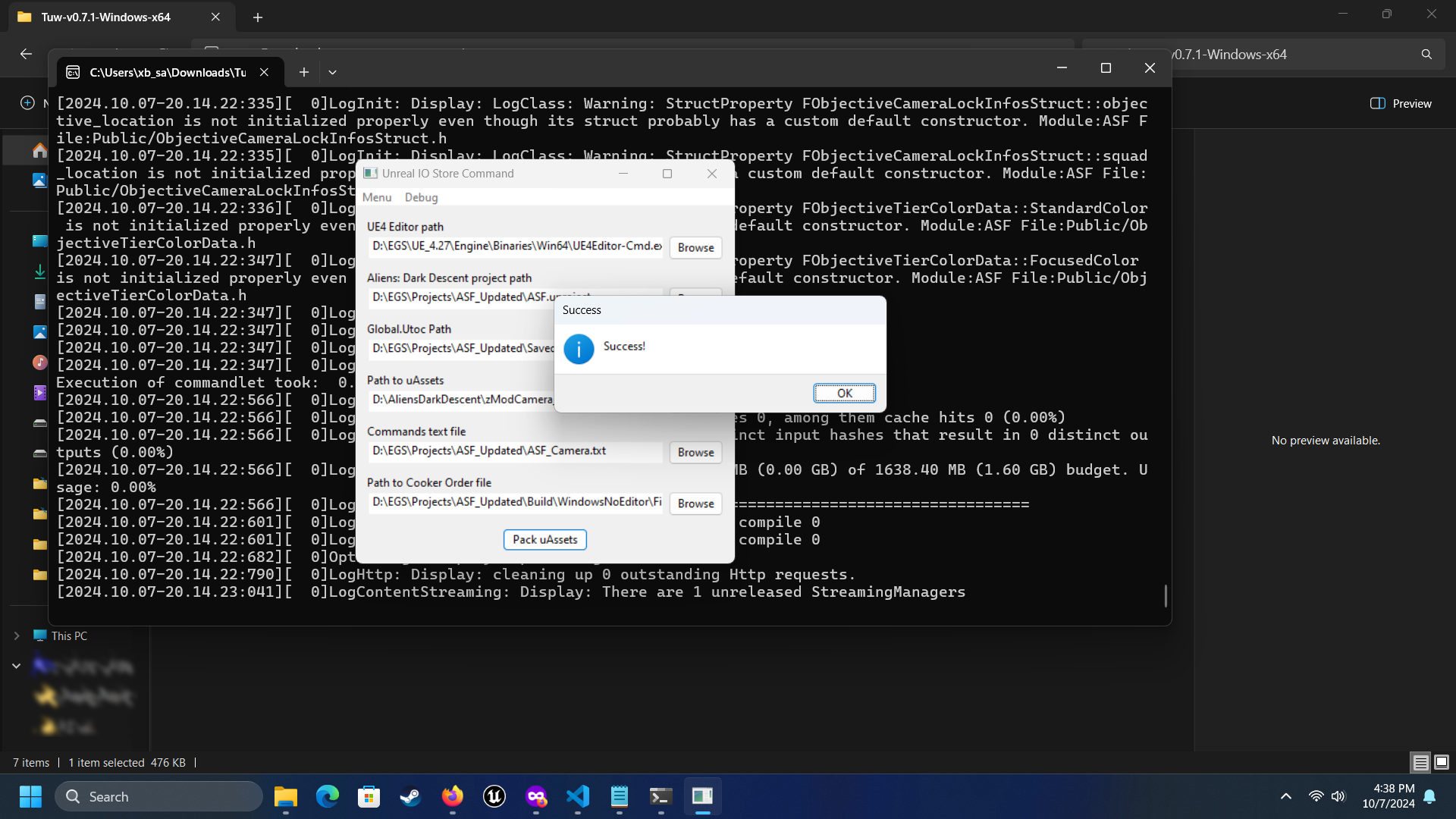
Tuw successfully completed the file generation part of the task, which meant any issues would be on my end.
The Cybersecurity Applications
How can this be leveraged for cybersecurity?
There's a non-zero use case for a GUI when you have a long command that requires multiple file and/or folder paths. But if we're honest, I doubt that it's all that common, and you're better off using a conventional script... unless your organization just blanket disables command line and PowerShell. Then this might be your only option.
But what comes to mind is a stupid simple way to execute troubleshooting commands on an endpoint PC for a non-technical and/or non-permissioned user. For example, you could make multiple Tuw based executable files for ping testing when a user calls with a network connection issue. One file would execute ping 8.8.8.8
, another would ping a specified endpoint, and so on, and each would only require the user to make a few clicks.
Naturally, you'd need to have someone qualified inspect the source code and verify that it was safe to use, but on the plus side, all those JSONs for your GUIs should be compatible across versions. If there's ever a problem, you can just rebuild the embedded GUI executables by combining a known good version of Tuw with those JSONs.